xuebaunion@vip.163.com
3551 Trousdale Rkwy, University Park, Los Angeles, CA
留学生论文指导和课程辅导
无忧GPA:https://www.essaygpa.com
工作时间:全年无休-早上8点到凌晨3点
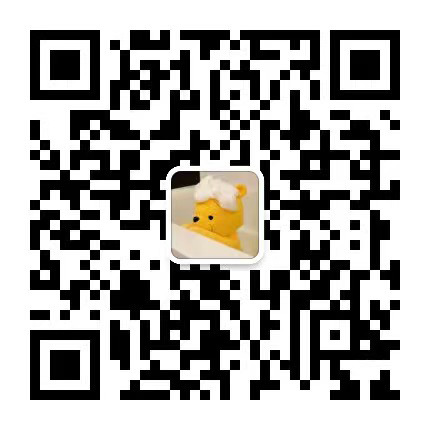
微信客服:xiaoxionga100
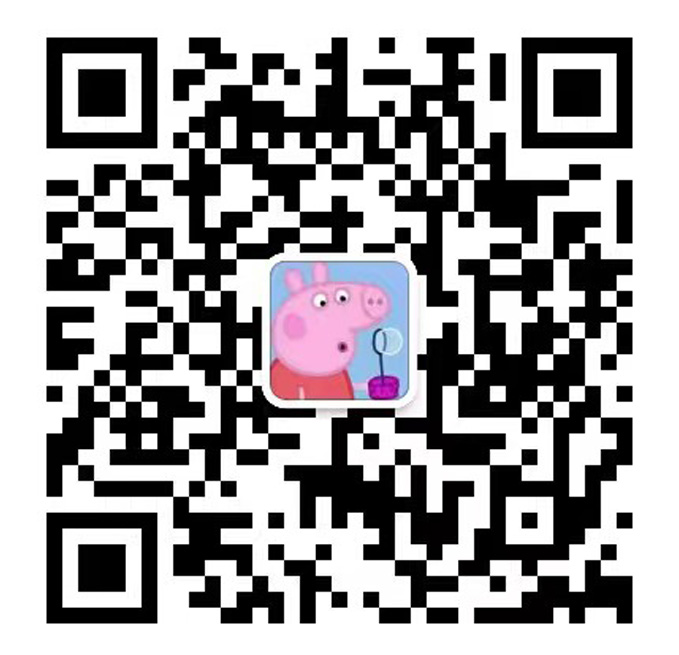
微信客服:ITCS521
This assignment assumes you have read the sections of Sobell specified on the course website. You should find the notes, and the two bash script references from the notes handy as well.
You must use your rlogin account or your own installation of linux when you analyze these questions. In all cases, your answers will be tested on the CentOS environment on rlogin.
You may work in pairs for this assignment. If you choose to work with a partner, make sure only one of you submits a solution, and that names and PIDs for both of you are listed as described below.
For question 1 type your answers in a plain text file named HW03.txt ; put the names and PIDs of both partners at the beginning of this file. For each of questions 2 and 3, you will write a single bash shell script; be sure to name your scripts exactly according to the instructions in the questions.
When you have completed the assignment, you will create an uncompressed tar file containing your HW03.txt file and
completed script files piggy.sh and piggy2.sh , and nothing else. Submit your file to the Curator system by the posted deadline for this assignment. No late submissions will be accepted.
You will submit your answers to the Curator System (www.cs.vt.edu/curator) under the heading HW03.
#! /bin/bash
#
# File: F.sh
F() {
if [[ $# -ne 2 ]]; then
echo "### error message 1 would be written from here"
exit -1;
fi
if [[! -d "$1" ]]; then
echo "### error message 2 would be written from here"
exit -2;
fi
name="$2".tar
cd "$1"
if [[ -e $name ]]; then
rm -f $name
fi
tar -cf $name *.h *.c
if [[ $? -ne 0 ]]; then
echo "### error message 3 would be written from here"
exit -
fi
echo "Contents:"
tar -tf $name
chmod 600 $name
}
exit $?
a) [5 points] What message would be most appropriate for "error message 1"? Justify your answer.
b) [5 points] What message would be most appropriate for "error message 2"? Justify your answer.
c) [5 points] What message would be most appropriate for "error message 3"? Justify your answer.
d) [10 points] Describe what you could accomplish by using this script.
Do not give a "blow by blow" description of the steps in the script! You can answer this question with one or two
sentences.
Testing a shell script is not very different from testing any program. You must create appropriate input; thats often a matter of setting up a directory structure containing a particular arrangement of files, with certain properties (e.g., permissions, file types, timestamps). Here are some suggestions:
Make liberal use of echo statements in testing your script. The contents of a string may not be at what you expect it to be, due to the effect of special characters. You may also find it useful to experiment with the use of single- and double-quotes, both in echo statements and elsewhere. Be creative in designing your test cases, but dont worry about handling every kind of error a user might make when writing command-line argument (at least not for this assignment). Read the man page for the touch and date commands. Its very handy, especially for question 3 below, to know how to manipulate the timestamps associated with a file. Be sure to disable diagnostic output in the release version (i.e., the one you submit) of your script.
A shell script is a program, and therefore subject to many of the same design and documentation expectations as any program you would write for an assignment in any course. In particular:
A script should check its command-line arguments, if it expects any. That includes not only making sure the right number of arguments are provided, but also checking for logically necessary preconditions (e.g., that a file does exist, or is readable). A script should be implemented using functions for actions that are likely to be useful in other scripts. (But, for this assignment, do not put those functions into an external file.) A script should not leave a trail of side-effects. For example, it should not alter any preexisting files unless the description of the scripts functionality implies it will do so. In some cases, a script may need to create a temporary file or directory to use during its execution; if so, the script should remove those things before it exits (in all cases). Whenever the script terminates, after a successful execution or otherwise, the script should print useful diagnostic messages to standard output. Such messages should be concise but informative. The script should be documented. Internal documentation serves two purposes. Major sections of the script should be preceded by a brief comment stating what role the section plays in the operation of the script. Within a section, comments serve to explain anything subtle that a casual user of the script might not easily understand. It is not necessary to write a comment for every line in the script; trivial comments (e.g., increment variable) actually reduce readability while providing no enlightenment.
The scripts you write for the following problems are expected to meet the expectations stated above.
Pig Latin is a game or a constructed language, take your pick. The rules we will use for constructing Pig Latin are:
Words that begin with a consonant or cluster of consonants have that initial consonant (or cluster) moved to the end
and followed by "ay":
Linux inuxLay
script iptscray
Words that begin with a vowel simply have "way" suffixed:
input inputway
argument argumentway
There are other sets of rules for constructing Pig Latin. For example, some schemes say that the second rule is applied to words that begin with a silent letter. For example, this would translate "knife" to "knifeway". That, however, is harder to program since it depends on pronunciation rules, and the same letter may be silent at the beginning of some words and not silent at the beginning of others. So, we will keep it simple and use the two rules given above.
# Synopsis: piggy.sh arg1 arg2 arg...
#
# Description:
# The given arguments are displayed to standard output in Pig Latin.
For example, the script might be invoked as:
CentOS > piggy.sh The Ohio State University
eThay Ohioway ateStay Universityway
CentOS > piggy.sh To be or not to be
oTay ebay orway otnay otay ebay
# Synopsis: piggy2.sh < filename
#
# Description:
# The file is parsed into strings, separated by whitespace, and those
# strings are translated into Pig Latin and written to standard output.
For example, the script might be invoked as:
CentOS > cat Hamlet.txt
to be or not to be
that is the question
CentOS > piggy2.sh < Hamlet.txt
otay ebay orway otnay otay ebay
atthay isway ethay uestionqay
# Synopsis: howmany dirname [ext]
# dirname must name a readable directory
# ext if present, is used as a file extension in matching
#
# Prints the number of regular files in the specified directory; if the
# invocation includes an extension (e.g. txt), reports only how many
# regular files match that extension.
#
# Exit codes:
# -1 wrong number of command-line parameters
# -2 dirname does not name a directory
# -3 dirname names an unreadable directory
For example, the script might be invoked as:
CentOS > ls -l Downloads/
total 20548
-rw-rw-r--. 1 wdm wdm 5120000 Apr 30 09:18 2505Current.tar
-rw-rw-r--. 1 wdm wdm 9195520 Apr 30 09:19 2506Current.tar
drwxrwxr-x. 4 wdm wdm 4096 Apr 3 22:40 C
-rw-rw-r--. 1 wdm wdm 92160 Apr 30 09:24 Cbackup.tar
-rw-rw-r--. 1 wdm wdm 4341760 Apr 30 09:26 mossbackup.tar
-rw-rw-r--. 1 wdm wdm 2284033 May 15 21:57 splint-3.1.2.src.tgz
CentOS > howmany.sh Downloads/
5
CentOS > howmany.sh Downloads/ tar
4
Aside from the course notes, the following may be useful:
Bash Guide for Beginners 8.2.1 the read built-in command and its use in reading from standard input 10.3.1 arithmetic operations on variables 11.1-3 functions in scripts
Advanced Bash-Scripting Guide 10.1 the eval built-in command and its use in manipulating strings