xuebaunion@vip.163.com
3551 Trousdale Rkwy, University Park, Los Angeles, CA
留学生论文指导和课程辅导
无忧GPA:https://www.essaygpa.com
工作时间:全年无休-早上8点到凌晨3点
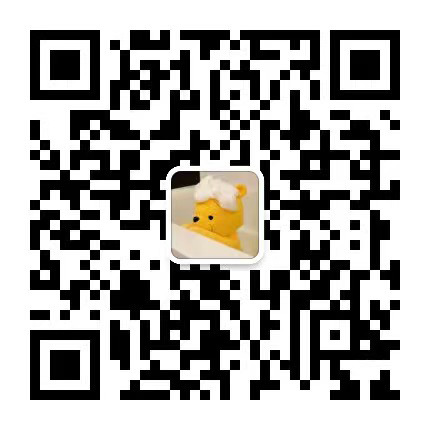
微信客服:xiaoxionga100
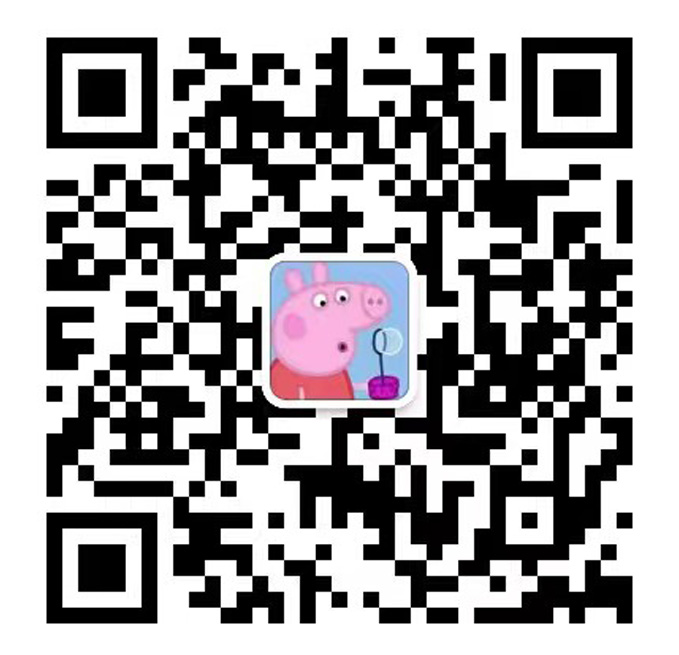
微信客服:ITCS521
MET CS521, Boston University, Fall 2021 Prof. Alan Burstein Homework 2 Due: Thursday, September 30th, 11:59PM EST 1 Without Coding Problem 1 (7 points) Rewrite this following "for" loop as a "while" loop: for i in range(1, X+1): if X % i == 0: print(i) [1] Problem 2 (7 points) What is the output of the following code? Show your work, including how x, i, and j change as the code runs. i = 0 j = 0 while i<10: for x in range(3): j+=2 i+=1 print(i,j) [2] Problem 3 (10 points) Briefly explain the following errors and an example that could cause them: • ZeroDivisionError • TypeError • NameError • SyntaxError • IndexError 1 of 4 MET CS521, Boston University, Fall 2021 Prof. Alan Burstein Problem 4 (6 points) What is the value of lines 2, 4, 5, 6, 9, and 11? Assume the lines are executed in order and errors may occur (as if they are separate notebook cells). 1. d = {’apples’ : 15, ’ bananas’: 35, ’ grapes’:12} 2. d[’banana’] 3. d[’oranges’]=20 4. len(d) 5. ’ grapes’ in d 6. d[’pears’] 7. fruits = list(d.keys()) 8. sorted( fruits ) 9. print( fruits ) 10. del d[’apples’] 11. ’ apples’ in d 2 Coding Problems Problem 5 (10 points) Write a letter grade method. It will take an input of a float and return the letter string. Score Letter 93+ A 90-93 A- 87-90 B+ 83-87 B 80-83 B- 77-80 C+ 73-77 C 70-73 C- 50-70 D 0-50 F The method signature should be calculate_letter (score). If a score is borderline, give it the higher letter grade. 2 of 4 MET CS521, Boston University, Fall 2021 Prof. Alan Burstein Problem 6 (10 points) Write a method to check if a year is a leap year. The method signature should be is_leap(year) and should return either True or False. To calculate if a year is a leap year, it must be divisible by 4, but NOT divisible by 100, UNLESS it’s divisible by 400. Problem 7 (10 points) Write a method to check if a number is a triangular number. The method signature should be is_triangle (num) and return either True or False. It is not trivial to check whether a number is triangular, but we can easily generate them using the formula: Tn = 1 + 2 + . . . + n Problem 8 (10 points) Write a method to find the sum of all the triangular numbers in an inclusive range. The method signature should be triangle_sum(lower_bound, upper_bound) and return the integer sum. You must also call is_triangle (). Problem 9 (10 points) Write a method that will return a list of random digits (1-9) of length n The method signature should be random_gen(n) and return a list of random numbers. You must use a loop, appending to a list, and the random module (specifically use random.randint). Your code may only call randint n times and cannot otherwise use the random module (extra calls will break the autograder). Problem 10 (10 points) Write a method digit_sum() that will add the digits of a given input and if the sum is a single digit, it will return that output and if not, it will add the digits of the following sum until a single digit sum is reached. The method signature should be digit_sum(num) and return an integer. Ex: digit_sum(129) = 3 9+2+1 = 12 × 1+2 = 3 X digit_sum(825) = 6 8+2+5 = 15 × 1+5 = 6 X digit_sum(11) = 2 1+1 = 2 X 3 of 4 MET CS521, Boston University, Fall 2021 Prof. Alan Burstein Submission Instructions Submit 2 files: hw2.py - For the coding part hw2.pdf - For the written part Submit both parts on Gradescope. At the top of hw2.pdf, include the following information: • Your name • The name of any classmates you discussed the assignment with, or the words "no collabora- tors" • A list of sources you used (textbooks, wikipedia, research papers, etc.) to solve the assign- ment, or the words "no sources" • Number of late days used for the current assignment, and the total number of late days used up thus far in the semester (including with the current assignment) Grading Methodology The written part of the assignment will be graded for correctness. In the case that your answer is incorrect, partial credit may be awarded based on the provided work. The code part of the assignment will be graded by an automatic grading program. It will use the method signatures specified in the assignment and use multiple test cases. 10 points are allocated for proper comments and styling and edge case detection: • Descriptive variable names • Comments for what functions do • Comments for complex parts of code • Proper naming conventions for any Variables, Functions, and Classes 4 of 4