xuebaunion@vip.163.com
3551 Trousdale Rkwy, University Park, Los Angeles, CA
留学生论文指导和课程辅导
无忧GPA:https://www.essaygpa.com
工作时间:全年无休-早上8点到凌晨3点
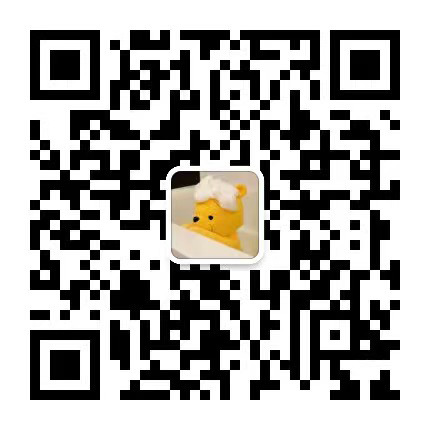
微信客服:xiaoxionga100
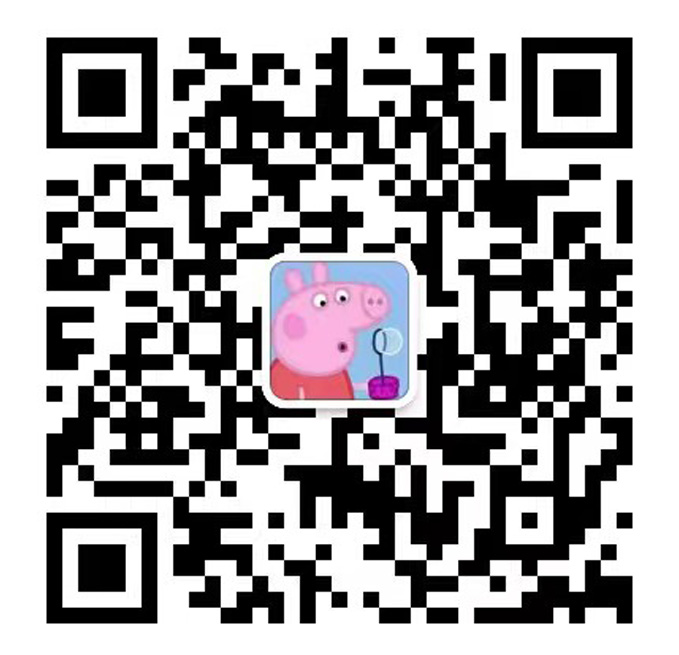
微信客服:ITCS521
This assignment has just one exercise. It is designed to help you get familiar with hashing and Java’s
HashMap.
You will need the following files:
Students.txt
Marking Scheme
Exercise 1 /22
• Working code, Outputs included, Efficient, Good basic comments included: 22/22
• No comments or poor commenting: subtract up to 3 points
• Unnecessarily inefficient: subtract up to 2 points
• No outputs and/or not all test cases covered: subtract up to 4 points
• Assignment submission and test case text files are not well labeled and organized: subtract
up to 2 points.
• Code not working: subtract up to 11 points depending upon how many classes and methods
are incorrect.
Error checking: Unless otherwise specified, you may assume that the user enters the correct data types
and the correct number of input entries, that is, you need not check for errors on input.
Submission: All submissions are through Brightspace. Log on dal.ca/brightspace using your Dal NetId.
Java Versions: Please ensure your code runs with Java 11.
What to submit:
Submit one ZIP file containing all source code (files with .java suffixes) and a text documents
containing sample outputs. For each exercise you will minimally have to submit a demo Class with a
main method to be tested and your test code with sample outputs.
Your final submission should include the following files: Exercise1.java, Students.txt,
Exercise1out.txt, and any other files needed to run your code.
Exercise1:
This exercise is designed to help you get familiar with the HashMap structure provided as part of the
Java standard libraries (https://docs.oracle.com/javase/7/docs/api/java/util/HashMap.html).
Write a program called Exercise1.java, that implements a simple, simulated login system using a pair
of HashMaps. Your program should accept input from a user (specifying the name of an input file)
and read a file formatted like the one described below (see also: Students.txt).
The input file will contain an arbitrary number of lines of user data. A user’s full name (first and
last), username, and password will be separated by tabs.
Ichabod Crane icrane qwerty123
Brom Bones bbones pass456!
Emboar Pokemon epokemon password123
Rayquazza Pokemon rpokemon drow456
Cool Dude cdude gh456!32
Trend Chaser tchaser xpxo567!
Chuck Norris cnorris power332*
Drum Dude ddude jflajdljfped
Capture data from the input file, creating 2 HashMaps:
1. A HashMap with username as key and the password as value.
2. Another HashMap with the username as key and full name as value.
Note: This is a toy program. It is acceptable to store the passwords in plaintext.
Now we’re going to create a simple login system with the stored info from the text file acting as the
password/login database.
After reading the input file and building the HashMaps, your program should prompt the user to enter
a login (username) and password. If the login is successful, print a welcome message. If the password
or username is incorrect, give the user 2 more chances. After 3 unsuccessful login attempts, your
program should print a failure message and exit. You will use the HashMaps to verify the password
entered by the user.
Test your program with a variety of inputs to ensure its proper operation. Do not hard code inputs. Save
data from your tests in a text file called Exercise1out.txt.
A sample run of your Exercise1.java program should look like this:
Login: rpokemon
Password: drow456
Login successful
Welcome Rayquazza Pokemon
A negative case should look like this:
Login: cdude
Password: trythis
Either the username or password is incorrect. You have 2 more attempts.
Login: cdude
Password: trythat
Either the username or password is incorrect. You have 1 more attempt.
Login: cdude
Password: 675rtht!
Sorry. Incorrect login. Please contact the system administrator.
Test Cases
Run your code against Students.txt. Show the following examples in the following order:
1. A case where the login is successful on 1 attempt
2. A case where the login is successful on the 2nd attempt
3. A case where the login is successful on the 3rd attempt
4. A case where the login is unsuccessful due to too many attempts
5. A case where the user can not be found (incorrect login info)
*Note: In all test cases, never use any real-world passwords or logins.
Save your test cases in a file called Exercise1out.txt.